c#
C# 배열 복사
1. Array.CopyTo(Array destArray, int index) // srcArray의 데이터를 인자로 전달한 destArray에 저장한다. // destArray의 마지막 인자의 인덱스 부터 저장된다. byte[] sourceArray = new byte[10]; // 복사를 할 배열 byte[] destinationArray = new byte[10]; // 복사를 당할 배열 // 복사 할 배열의 데이터를 삽입 for (int i = 0; i < 10; ++i) sourceArray[i] = (byte)i; // 복사하기전 복사를 당할 배열의 데이터를 출력 for (int i = 0; i < 10; ++i) Console.Write($"{destinationArray[i]} "); //..
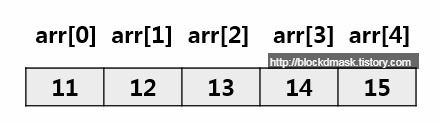
C# 배열 초기화, 다차원배열, 가변배열에 대해서
1. C# 배열의 선언 초기화 사용 방법 (Array) -> 배열이란 ? : 배열이란 관련있는, 비슷한 데이터를 효과적으로 관리하기 위한 자료구조다. : 배열을 이용하면 연관되어있는 데이터들을 for문과 결합하여 손쉽게 순회 할 수 있다. C#에서의 배열 선언 방법 1. 기본 모양 - 자료형[] 변수이름 = new 자료형[N] { 초기화 하거나 안하거나}; 2. 배열의 요소 개수를 지정하고 선언과 동시에 초기화 하는 방법 - int[] arr1 = new int[5] { 11, 12, 13, 14, 15 }; 3. 배열의 요소 개수를 지정하지 않고 선언과 동시에 초기화 하는 방법 - int[] arr1 = new int[] { 11, 12, 13, 14, 15 }; 4. 배열의 요소 개수를 지정하고, 추..
C# 제네릭 형식 제약 조건 (where)
제네릭 제약 조건 추가 class GenericClass { public T objMember { get; set; } } class Program { static void Main(string[] args) { GenericClass genericObj1 = new GenericClass(); GenericClass genericObj2 = new GenericClass(); GenericClass genericObj3 = new GenericClass(); } } 제네릭은 모든 타입을 허용하는 기법이므로 GenericClass 클래스의 objMember 멤버 변수는 값 형식인 int형이 될 수도 있고 참조 형식인 string형과 ArrayList 타입도 가능하다. "GenericClass 클래스의 o..
C# 난수 생성 Random 클래스
1. C# random(랜덤) 클래스에 대해서. 랜덤 클래스가 정의 되어있는 곳을 살펴보면 아래 캡쳐와 같다. 1. 랜덤클래스는 System 네임스페이스 안에 속해있다. Random 클래스는 namespace System 괄호 안에 존재한다. Systen 네임스페이스는 C# 파일을 만들면 자동으로 포함되어있기 때문에 Random 클래스를 사용하기 위해서 특별히 무언가를 추가한다거나 할 필요가 없다. 2. 랜덤클래스 객체를 생성하는 방법 (생성자) Random 변수명 = new Random();: new 키워드와 생성자를 이용해서 Random 타입의 객체를 생성한다. 3. 랜덤클래스의 Next() 메서드 Next() 메서드는 임의의 정수를 반환하는 메서드(멤버 함수) 입니다. (음수는 나오지 않음, n>=..
C# 구조체가 IEquatable<T>를 상속해야 하는 이유
C#의 모든 타입의 최상위 클래스인 Object에는 .Equals(object) 메소드가 존재한다. 그리고 이를 통해 다른 값과의 동일 비교를 수행할 수 있다. 구조체나 클래스를 직접 정의하고, 해당 객체로 .Equals(object)를 호출하면 object.Equals(object)가 호출된다. 박싱 int a = 1; object oa = a; Value Type을 object 타입으로 캐스팅하면 박싱이 일어난다. IL_0001: ldc.i4.1 IL_0002: stloc.0 IL_0003: ldloc.0 IL_0004: box [mscorlib]System.Int32 IL_0009: stloc.1 디스어셈블러를 통해 CIL 코드를 열어보면 위와 같이 확인할 수 있다. .Equals(object)에서..
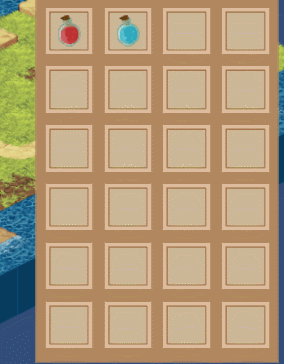
[Unity] EventSystem을 이용해 아이템UI 드래그 및 다른 슬롯에 등록하기(IDragHandler, IDropHandler)
드래그, 드랍할 UI오브젝트에 Drag관련 인터페이스가 포함된 스크립트를 추가 public class ItemDragHandler : MonoBehaviour, IBeginDragHandler, IDragHandler, IEndDragHandler 그리고 인터페이스에 해당하는 함수를 구현해주면 된다. 드래그해서 마우스를 따라다니게 하고 싶으므로 IDragHandler의 OnDrag함수를 구현해주면 된다. public void OnDrag(PointerEventData eventData) { transform.position = eventData.position; } 참고로 eventData.position 말고 Input.mousePosition 을 써도 된다. 아이템을 놓았을 때 제자리로 돌아가게 하고..
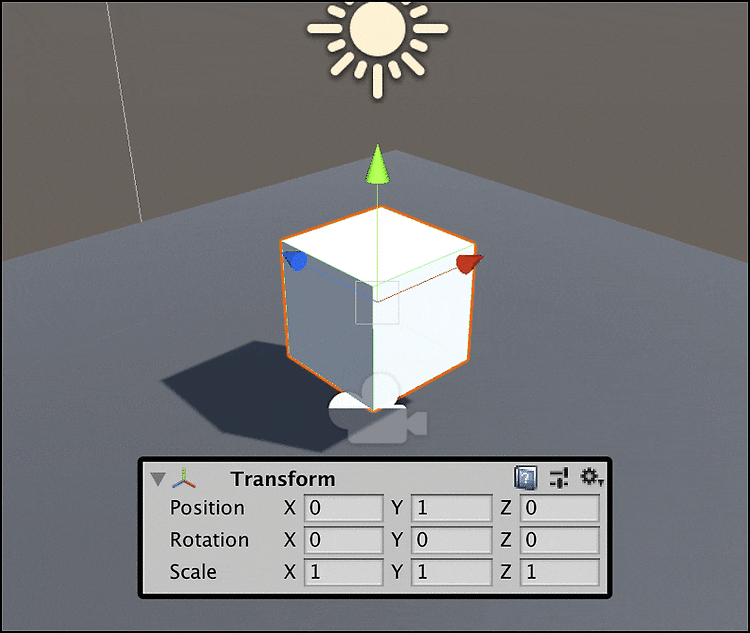
[Unity] 게임 오브젝트 컴포넌트 패턴 (GameObject) C# 구현
아래는 간단하게 구현해본 컴포넌트 패턴이다, gameObject라는 매개체 즉 자기 자신을 통해 해당 오브젝트가 존재하는지 확인 및 추가 기능. using System; using System.Collections.Generic; namespace ConsoleApplication1 { public class GameObject { // 컴포넌트 리스트 public List lstComp = new List(); public T GetComponent() where T : Component { // 리스트가 해당 오브젝트를 가지고 있을 시 if (lstComp.Contains(typeof(T))) { Type type = lstComp[lstComp.IndexOf(typeof(T))]; T comp = ..
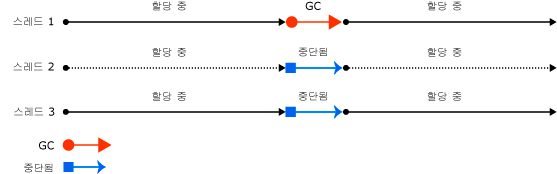
C# 가비지 컬렉터 (Garbage Collector / GC)
GC가 작동하는 시기 객체를 할당하여 할당하는 임계치가 넘어갈 때 (각 세대 별) 시스템의 메모리가 부족할 때 GC.Collect 메서드를 호출할 때 GC Root 루트는 힙에 있는 최상위 객체를 가리키는 참조를 말한다 스택이나 힙(static)에 생성된다. .NET 어플레이케이션을 실행하면, JIT 컴파일러가 루트 목록을 생성하고 CLR이 루트 목록을 돌면서 상태를 갱시하는 것이다. (GC가 참조함) 가비지 컬렉터는 루트 목록을 순회하면서 루트가 참조하는 힙 객체와 관계를 조사한다. 어떤 힙과도 루트와 관계가 없다면 필요 없는 Garbage 다른 힙 객체를 참조한다면 Not Garbage 쓰레기 객체가 있던 메모리는 비워줘야 한다.(Sweap) 이 때문에 이러한 방법의 특징은 Heap 전체를 검사할 필..
64bit OS에서 C++과 C# 데이터 차이 비교
CTS C++ C# Size(byte) System.Byte unsigned char byte 1 System.SByte signed char sbyte 1 System.Int16 short short 2 System.UInt16 unsigned short ushort 2 System.Int32 int int 4 System.Int64 long, long long long 8 System.Single float float 4 System.Char wchar_t char 2 System.Double double double 8 System.UInt64 size_t ulong 8 System.IntPtr void * (pointer) IntPtr 8 출처 : https://dragontory.tistory...
C# ILookup과 Lookup<TKey, TElement>와 Dictionary<TKey, TValue>간 차이
Dictionary 는 키를 단일 값에 매핑하는 반면 Lookup 는 키를 값 컬렉션에 매핑한다. 값 컬렉션이라고 하면은 LINQ에서 반환되는 모든 값들을 뜻한다고 볼 수가 있다. 아래는 Type 리플렉션을 사용한 예제이다. using System; using System.Collections.Generic; using System.Runtime.InteropServices; using System.Linq; using System.Xml; namespace ConsoleApplication1 { public class Test { static void Main() { // Type형 배열에 List 타입, string 타입, Enumerable 타입 그리고 XmlReader 타입 추가 Type[] ar..