C++
DirectX 11 LNK2019 에러 해결 방법
Unresolved external symbol __vsnprintf .... (in dxerr.lib) #pragma comment(lib, "legacy_stdio_definitions.lib") d3dUtil.h 헤더파일 내 HR 매크로 오류 #pragma comment(lib, "dxerr.lib") #pragma comment(lib, "D3DX11.lib")
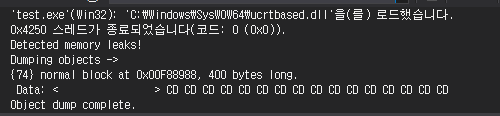
RAII (Resource Acquisition Is Initialization)
RAII는 Resource acquisition is initialization의 약자로 C++설계 패턴중 하나인 키워드이며 흭득된 자원을 초기화 한다. 동적인 프로그래밍을 위해 new라는 키워드를 사용해 힙 메모리에서 할당받는다. 할당 받는순간 해당 메모리의 resource를 프로그래머는 직접 관리하게 된다. 예기치 못한 exception등... 다양한 이유로 인해 할당받은 메모리를 해제하지 못하고 Memory leak이 발생하게 된다. 뿐만 아니라 mutex의 lock에서도 발생할 수 있다. 이러한 문제들을 안전하게 관리하고자 만든 것들이 unique_ptr, shared_ptr, lock_guard 등...이 있다. 해당 클래스들은 함수가 끝나면, {}(중괄호)에서 벗어 난다면.... finally..

C++ 문자열 공백 제거하는 방법
#include #include #include #include #include #include #include #include using namespace std; int main() { string str = "Hello World!"; string str2 = str, str3 = str; cout
[실1] 14888 - 연산자 끼워넣기
#include using namespace std; #define OPERATOR_COUNT 4 int n; int arr_operand[1000]; // 수열 int arr_operator[OPERATOR_COUNT]; int min_val = INT_MAX; int max_val = INT_MIN; void DFS(int result, int idx) { if (idx == n) { if (result > max_val) max_val = result; if (result 0) { arr_operator[i]--; ..
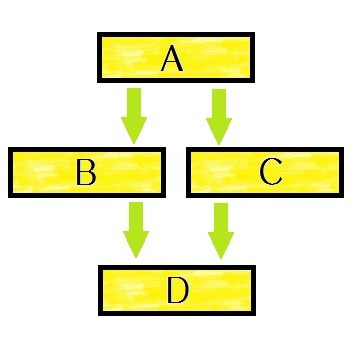
C++ virtual 다중 상속, 가상 부모 클래스
다중 상속을 할 시 예상치 못하게 클래스가 중복될 가능성이 있다. class A { public: int a; }; class B : public A { public: int b; }; class C : public A { public: int c; }; class D : public B, public C { public: int d; }; A 클래스를 B, C 클래스가 상속받고 B, C 클래스를 D클래스가 상속받는 구조다. 문제는 이와같은 구조로 상속받을 때 A클래스의 내용물이 중복이 된다 하지만 중복되는 걸 희망하지 않을 때는 virtual 상속을 사용하면 된다. 클래스를 상속받을 때 상속받는 클래스에 virtual 을 앞에 붙여주면 된다 그렇게 되면, B, C 클래스를 상속받는 경우 B, C 클래스..
[실1] 11403 - 경로 찾기
플로이드 와샬 #include #define INF 100000 #define NODE 1000 using namespace std; int graph[NODE][NODE]{ 0 }; void Floyd_washall() { int n; cin >> n; for (int i = 0; i > graph[i][j]; } for (int k = 0; k < n; k++) // 거쳐가는 노드 { for (int i = 0; i < n; i++) // 출발지 노드 { for (int j = 0; j < n; j++) // 도착지 노드 { if (graph[i][k] && graph[k][j]) graph[i][j]= 1; } } } ..
컴파일 속도 향상 (시간 초과 오류)와 Stream (스트림)
ios::sync_with_stdio, cin.tie, cout.tie란? ios::sync_with_stdio(0); cin.tie(0); cout.tie(0); Stream 우선 stream에 대한 이해가 먼저 필요하다 우리가 c언어와 c++언어를 가장 처음 배울 때 적는 것은 사실 아래에 두 헤더파일이다 각각은 stdio: standard input output iostream: input output stream #include #include 표준 스트림(standard streams)은 특정한 프로그래밍 언어 인터페이스뿐 아니라 유닉스 및 유닉스 계열 운영 체제(어느 정도까지는 윈도에도 해당함)에서 컴퓨터 프로그램과 그 환경(일반적으로 단말기) 사이에 미리 연결된 입출력 통로를 가리킨다. 우리..
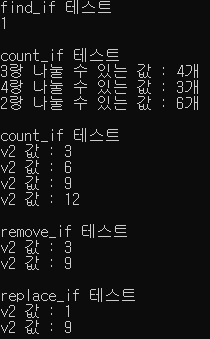
C++ _if STL 알고리즘 함수 (파라미터 _Pr _Pred) > 조건자 (Predicate)
참고할만한 부분은 _if로 끝나는 함수들만 매개변수(조건자)로 넘길 수 있다는 점, sort 함수 예외. 참고 사이트 : https://en.cppreference.com/w/cpp/algorithm #include #include #include #include #include using namespace std; bool Is_one(int _val) { return _val == 1; } class Test { public: bool operator()(int i) { return i % 4 == 0; } }; struct s_test { public: bool operator()(int i) { return i % 2 == 0; } }; int main() { // 파라미터 _Pr _Pred > ..
[실1] 11286 - 절대값 힙
#include #include #include #include using namespace std; #define y first #define x second int main() { using pq_type = pair; cin.sync_with_stdio(0); cin.tie(0); priority_queue q; int n; cin >> n; for (int i = 0; i > val; if (val != 0) q.push({ abs(val), val }); else { if (q.empty()) cout
C++ 구조적 바인딩 (Structured Bindings)
C++17부터 지원하는 structured binding이란 어떤 배열, STL 같은 컨테이너에서 멤버들을 쉽게 바인딩할 수 있도록 도와주는 문법이다. 친숙한 std::map을 예시로 바인딩을 해보자. #include #include int main() { std::map mp; for (int i = 1; i < 11; i++) { mp[i] = i * i; } // iterator, basic for for (std::map::iterator it = mp.begin(); it != mp.end(); it++) { std::cout first