개념
부모 클래스 내 함수를 자식 클래스 재정의 하는 것이며 C++하곤 살짝 다른 개념이다 기본적으로 룰이 존재하는데.
1. 절대로 private이면 안된다.
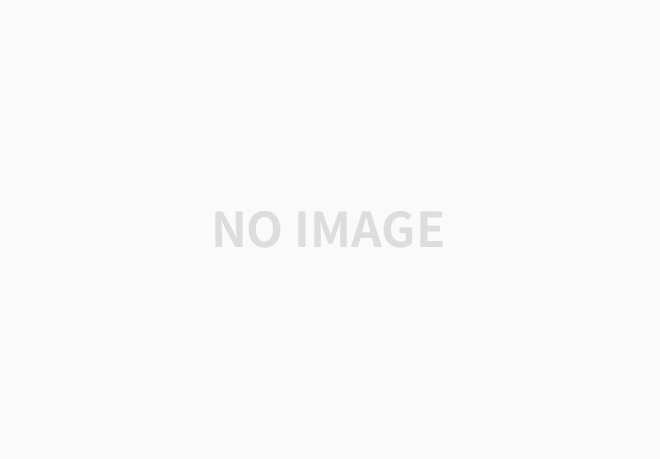
2. C++과 다르게 같은 함수명을 적어도 컴파일러는 알아채질 못한다.
using System;
using System.Collections.Generic;
using System.Runtime.InteropServices;
namespace ConsoleApplication1
{
public class Parent
{
int mVal = 0;
public int ValProp
{
get { return mVal; }
set { mVal = value; }
}
public Parent()
{
this.mVal = 10;
Console.WriteLine("기본 생성자");
}
public virtual void Print()
{
Console.WriteLine("부모 Print 함수");
}
}
public class Child : Parent
{
public void Print()
{
Console.WriteLine("자식 Print 함수");
}
static void Main()
{
Parent parent = new Child();
parent.Print();
}
}
}
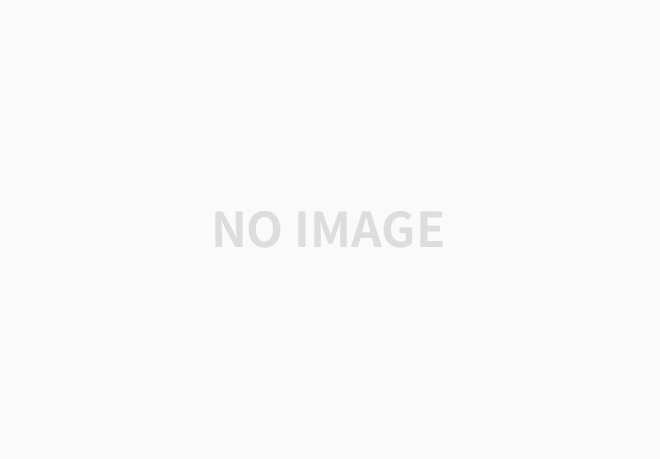
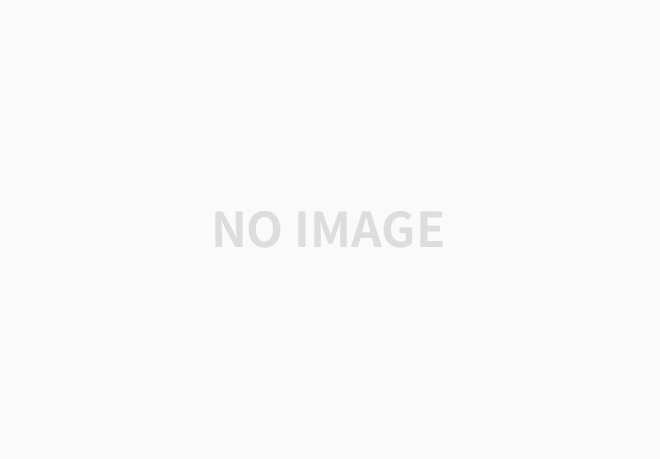
자식 클래스 내에 virtual 대신 override로 바꾸면 아래와 같이 정상 출력이 된다.
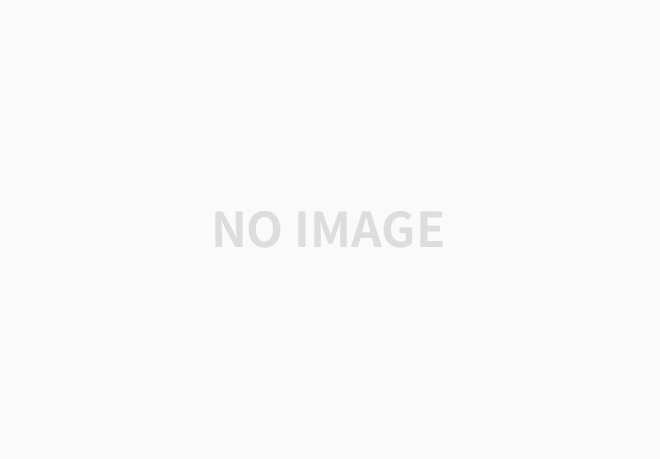
3. C++과 다르게 접근자 또한 일치해야한다.
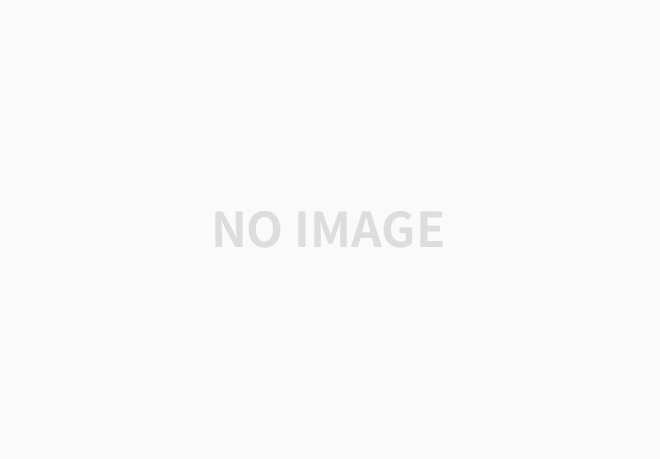
부모 함수 또는 생성자 호출
C++ 에선 부모 클래스명::으로 했다, C#에서는 static 함수로 인식하여 에러가 뜬다 따라서 base 키워드를 붙여야하며 대리 생성자 사용할 때도 자식 클래스명() : 부모 클래스명()가 아닌 base 키워드를 붙여줘야한다.
using System;
using System.Collections.Generic;
using System.Runtime.InteropServices;
namespace ConsoleApplication1
{
public class Parent
{
int mVal = 0;
public int ValProp
{
get { return mVal; }
set { mVal = value; }
}
public Parent()
{
this.mVal = 10;
Console.WriteLine("부모 기본 생성자");
}
public virtual void Print()
{
Console.WriteLine("부모 Print 함수");
}
}
public class Child : Parent
{
public Child() : base()
{
Console.WriteLine("자식 기본 생성자");
// 아래는 오류 static 메서드로 인식
// Parent.Print();
base.Print();
}
public override void Print()
{
Console.WriteLine("자식 Print 함수");
}
static void Main()
{
Parent parent = new Child();
parent.Print();
}
}
}
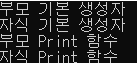
'프로그래밍 언어 > C#' 카테고리의 다른 글
C# 람다식 (lambda expression) (0) | 2022.08.06 |
---|---|
C# 가변 길이 배열 (Variable Length Array) (0) | 2022.08.02 |
C# 자기 자신 참조 (this) / 위임 생성자 (delegating constructor) (0) | 2022.07.28 |
C# 추상 클래스 (abstract) (0) | 2022.07.28 |
C# 인터페이스 (interface) (0) | 2022.07.28 |